In this
article:
Source Code
License
Navigation:
HomeHardware
Software
Techniques
Controllers
Reviews
Index
Description
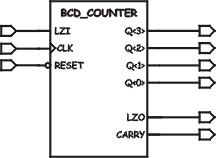
The image at right is the schematic symbol for the BCD_COUNTER module. Three inputs and six outputs.
It is a very simple module and by decomposing the counting into individual digit modules, the VHDL to specify the counter became significantly easier to write. There are many ways to do this of course, perhaps a sub procedure or a function statement but this worked well.
The interesting bit about this counter is that it implements “part” of the suppress leading zeros protocol. It does this by asserting LZO (Leading Zero Out) if the counter before it asserted it and it is currently holding zero.
The Source Code
-- BCD_COUNTER.VHD Chuck McManis 22-Mar-01 -- -- This module implements a simple divide by 10 (binary coded decimal) -- counter. It has the added feature that it asserts "LZO" (leading -- Zero Out) high if it is holding zero, and the "LZI" (leading zero -- in) pin is also high. Thus a cascade of these can indicate to -- external logic which digits should be blanked when leading zero -- supression is desired. -- library IEEE; use IEEE.STD_LOGIC_1164.ALL; use IEEE.NUMERIC_STD.ALL; use IEEE.STD_LOGIC_UNSIGNED.ALL; entity bcd_counter is Port ( clk : in std_logic; -- count input reset : in std_logic; -- resets count to 0 carry : out std_logic; -- Overflow (from 9) lzi : in std_logic; -- Leading Zero IN lzo : out std_logic; -- Leading Zero OUT q : out std_logic_vector(3 downto 0) ); end bcd_counter; architecture behavioral of bcd_counter is signal h : std_logic_vector(3 downto 0); begin -- Primary counting process, one 4 bit latch holds the -- current count. CTR: process(clk,reset) is begin if (reset = '0') then h <= "0000"; carry <= '0'; elsif (clk'event and clk = '1') then if ( h = 9) then h <= "0000"; carry <= '1'; else h <= h + 1; carry <= '0'; end if; end if; end process; -- Assign holdign register to the output pins. q <= h; -- Set the state of the LZO pin based on H and LZI lzo <= '1' when (lzi = '1') and (h = "0000") else '0'; end behavioral;
License
This work is licensed under a Creative Commons Attribution-NonCommercial 3.0 Unported License. You are free to play around with it and modify it but you are not licensed to use it for commercial purposes. Click the link above for more details on your rights under this license.